Hands on introduction to goLang (Go Language)
- Sunil Kumar Padmavathy
- Oct 20, 2016
- 2 min read
goLang (Go) is a free open source programming language created at Google in 2007 by Robert Griesemer, Rob Pike, and Ken Thompson The intention of creating a new language was to make use of the positive characteristics of existing languages while leaving out the negatives. The guideline was to make a new language which is scalable, which could be statically typed, be productive and readable, without too many keywords and repetitions, doesn't require specific IDE and support networking and multiprocessing.
Go is claimed to be more expressive, concise, clean and efficient programming language. Let's get a hands on with a simple program.
Step1: Different ways to execute the program
You may execute the below program in two ways.
a) Goto https://golang.org/ and execute the code in the 'Try Go' window.

b) Install Go.
Goto https://golang.org/dl/ and download the appropriate package and follow the installation process.
Set up the environment variable GOROOT by assigning the value as the folder location where Go is installed in your machine.
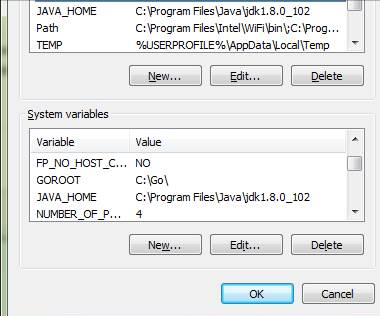
To validate if Go is installed, open command prompt (click windows button on bottom left hand corner and type cmd and click on the cmd.exe, now type go
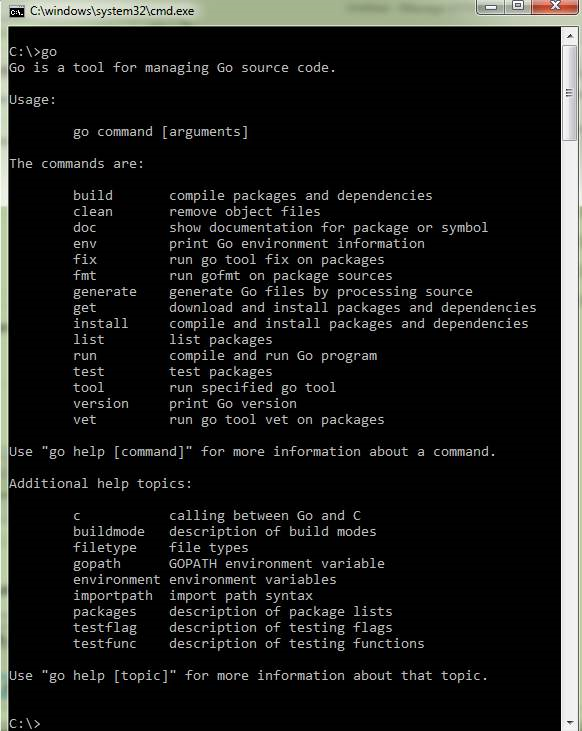
The above result means that Go is installed, you can also see that the gopath is configured here.
Now, we are ready to run the program using the Go compiler.
Step 2: Write and save the Go program
Now, Open a notepad or notepad++ and paste the below code
package main import "fmt" func main() { fmt.Println("Hello, Friend") //example 1: addition a:=1 b:=2 fmt.Println("added value is ",a+b) //example 2: subtraction var num1 int = 60 var num2 int= 30 fmt.Print("subtracted value is ",num2-num1) }
you might have noticed that unlike java and other programming languages, Go doesn't require us to add a semicolon(;) at the end of each line.
Now save the file as goTest.go
(you may give any name but it must end with .go extension)
Save it in a folder location.
Step 3: Navigate to the file path and execute the program
once you navigate to the file path, use the command
go run <file name> to run the program

you may see that
fmt.Println("Hello, Friend")
--> has printed a string value "Hello, Friend"
//example 1: addition a:=1 b:=2 fmt.Println("added value is ",a+b)
--> has printed the sum of a and b as 3
//example 2: subtraction var num1 int = 60 var num2 int= 30 fmt.Print("subtracted value is ",num2-num1)
--> has printed the difference of num2 and num1 as -30
For more details visit the below links. Enjoy learning!
References:
https://en.wikipedia.org/wiki/Go_(programming_language)
https://golang.org
Kommentare